[딥러닝초급]Custom Convnets
Introduction
Now that you've seen the layers a convnet uses to extract features, it's time to put them together and build a network of your own!
앞서 컨브이넷이 피쳐 추출을 사용하기 위한 레이어에 대해 공부를 했고, 이제 자신만의 신경망모델을 작성해보자.
Simple to Refined
In the last three lessons, we saw how convolutional networks perform feature extraction through three operations: filter, detect, and condense. A single round of feature extraction can only extract relatively simple features from an image, things like simple lines or contrasts. These are too simple to solve most classification problems. Instead, convnets will repeat this extraction over and over, so that the features become more complex and refined as they travel deeper into the network.
앞서 3개의 강의를 통해 우리는 컨볼루션 네트워크가 피쳐 추출을 위해 3가지 작업을 수행한다는 내용을 배웠다. 필터(filter) / 디텍트(detect) / 컨덴스(Condense) - FDC 피쳐 추출을 진행하면 처음에는 라인 종류나 콘트라스트 등의 단순한 피쳐들이 추출된다. 그러나 추출이 반복되면 피쳐는 좀 더 복잡해지고 신경망 내에 심층 학습을 통해 좀 더 정교해진다. (#당연한 이야기니 넘어가자. 중요한 것은 필터 / 디텍트 / 컨덴스)
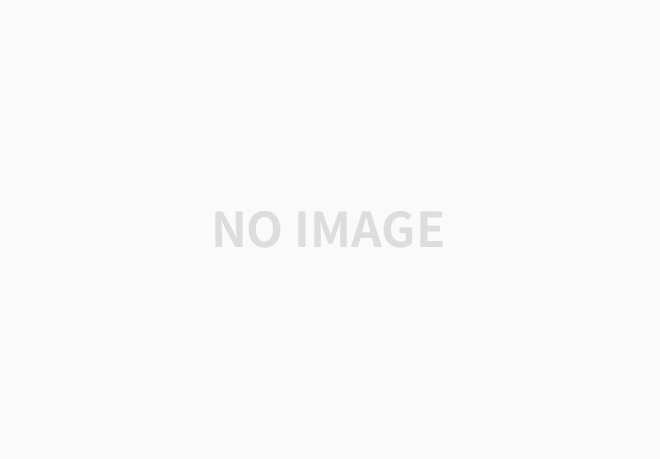
These convolutional blocks are stacks of Conv2D and MaxPool2D layers, whose role in feature extraction we learned about in the last few lessons.
이 컨볼루션 블록들은 Conv2D나 MaxPool2D와 같은 레이어들을 겹겹히 쌓아올린 것(stacks of). 그리고 목적은 피쳐 추출이다.
Each block represents a round of extraction, and by composing these blocks the convnet can combine and recombine the features produced, growing them and shaping them to better fit the problem at hand. The deep structure of modern convnets is what allows this sophisticated feature engineering and has been largely responsible for their superior performance.
각 블록은 피쳐추출의 한 라운드를 나타내며 이것들을 반복하고 엮어서 컨브이넷(Convnet)은 좀더 문제를 잘 풀수 있는 (better fit)모델에 접근하게 된다. 오늘날 컨브이넷이 갖는 성능상의 차별화는 이 복잡한 피쳐 엔지어링을 어떻게 잘 조합하고 더 높은 성능을 갖도록 조율하는 가에 달려 있다.
Example - Design a Convnet
Let's see how to define a deep convolutional network capable of engineering complex features. In this example, we'll create a Keras Sequence model and then train it on our Cars dataset.
컨볼루션 심층망의 복잡한 피쳐 엔지니어링을 정의해보자. 우리는 케라스의 Sequence 모델을 이용하여 Cars 데이터셋을 학습시킬 것이다.
Step 1 - Load Data
This hidden cell loads the data. 우선 데이터를 로드한다.
Step 2 - Define Model
Here is a diagram of the model we'll use: 아래는 우리가 사용하려는 모델을 나타낸 다이어그램이다.
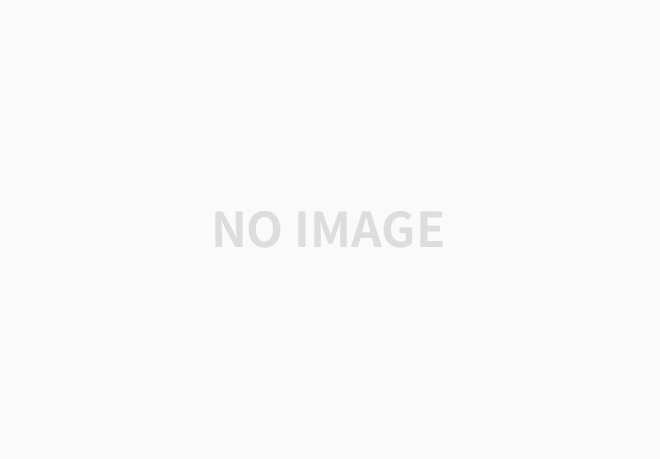
Now we'll define the model. See how our model consists of three blocks of Conv2D and MaxPool2D layers (the base) followed by a head of Dense layers. We can translate this diagram more or less directly into a Keras Sequential model just by filling in the appropriate parameters.
이제 모델을 정의할건데, 덴스 레이어(head) 전에 어떻게 Conv2D나 MaxPool2D 레이어를 베이스로 구성하는지 잘 보기 바란다. 우리는 다이어그램에 나온 모델을 케라스의 Sequential 모델로 바로 바꿔서 넣고 있고 각 파라미터만 조정하면 된다.
#파이썬 코드
from tensorflow import keras
from tensorflow.keras import layers
model = keras.Sequential([
# First Convolutional Block
layers.Conv2D(filters=32, kernel_size=5, activation="relu", padding='same',
# give the input dimensions in the first layer
# [height, width, color channels(RGB)]
input_shape=[128, 128, 3]),
layers.MaxPool2D(),
# Second Convolutional Block
layers.Conv2D(filters=64, kernel_size=3, activation="relu", padding='same'),
layers.MaxPool2D(),
# Third Convolutional Block
layers.Conv2D(filters=128, kernel_size=3, activation="relu", padding='same'),
layers.MaxPool2D(),
# Classifier Head
layers.Flatten(),
layers.Dense(units=6, activation="relu"),
layers.Dense(units=1, activation="sigmoid"),
])
model.summary()
Notice in this definition is how the number of filters doubled block-by-block: 32, 64, 128. This is a common pattern. Since the MaxPool2D layer is reducing the size of the feature maps, we can afford to increase the quantity we create.
이 정의에서 필터의 숫자는 블록별로 32, 64, 128인데 이것이 통상적인 패턴이다. MaxPool2D 레이어는 피쳐맵의 사이즈가 줄어들고 그 때문에 늘어난 위 숫자를 처리할 수 잇다.
Step 3 - Train
We can train this model just like the model from Lesson 1: compile it with an optimizer along with a loss and metric appropriate for binary classification.
이제 모델 트레이닝이 가능하다.
#파이썬코드
model.compile(
optimizer=tf.keras.optimizers.Adam(epsilon=0.01),
loss='binary_crossentropy',
metrics=['binary_accuracy']
)
history = model.fit(
ds_train,
validation_data=ds_valid,
epochs=40,
verbose=0,
)
import pandas as pd
history_frame = pd.DataFrame(history.history)
history_frame.loc[:, ['loss', 'val_loss']].plot()
history_frame.loc[:, ['binary_accuracy', 'val_binary_accuracy']].plot();
This model is much smaller than the VGG16 model from Lesson 1 -- only 3 convolutional layers versus the 16 of VGG16. It was nevertheless able to fit this dataset fairly well. We might still be able to improve this simple model by adding more convolutional layers, hoping to create features better adapted to the dataset. This is what we'll try in the exercises.
위의 결과는 Lesson 1 에서 단 3개의 컨볼루션 레이어를 가졌던 VGG16 모델 결과와 비교하면 훨씬 작은 값을 가질 거다. 결과로 보면 이 모델은 해당 데이터셋에 매우 잘맞는다고 할 수 있을 것이다. 그리고 아마도 우리는 좀 더 많은 컨볼루션 레이어를 더하여 더 정교한 모델을 만들 수도 있다.
연습문제에서 계속해보자.